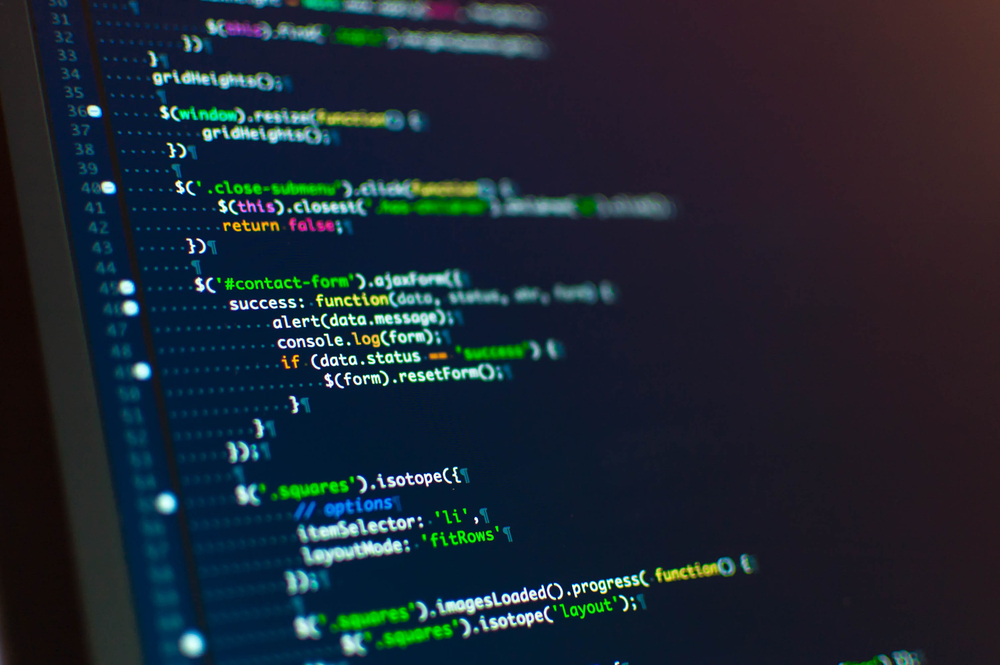
Here is a simple wrapper function I created for use with the SharePoint Rest API. If an Id is entered the function will update a record, otherwise it will insert a new record.
Usage:
[SyntaxHLNumbers]
//Create ListItem Object
var customers = {};
//Set List Name
customers._listName = “Customers”;
//Add columns to insert
customers.Name = “Michael Tippett”;
customers.Email = “My@email.com”;
//Insert into SharePoint
SpproJsFramework.listItem.upsert(customers, successDelegate, errorDelegate);
[/SyntaxHLNumbers]
Under the hood:
[SyntaxHLNumbers]
var SpproJsFramework {
listItem: {
upsert: function(listObject, success, failure) {
var itemType = this.GetItemTypeForListName(listObject._listName);
//Insert URL
var url = _spPageContextInfo.siteAbsoluteUrl + “/_api/web/lists/getbytitle(‘” +
listObject._listName + “‘)/items”;
if (listObject.Id) {
//Update not Insert
url = _spPageContextInfo.siteAbsoluteUrl + “/_api/web/lists/getbytitle(‘” +
listObject._listName + “‘)/items(” + listObject.Id + “)”;
}
var item = {
“__metadata”: {
“type”: itemType
}
};
//Add properties to rest object
for (var key in listObject) {
if (key.charAt(0) != “_”) {
item[key] = listObject[key];
}
}
var headers = {
“Accept”: “application/json;odata=verbose”,
“X-RequestDigest”: $(“#__REQUESTDIGEST”).val(),
};
if (listObject.Id) {
//Update (Merge) headers
headers = {
“Accept”: “application/json;odata=verbose”,
“content-type”: “application/json; odata=verbose”,
“X-RequestDigest”: $(“#__REQUESTDIGEST”).val(),
“X-HTTP-Method”: “MERGE”,
“If-Match”: “*”
}
}
$.ajax({
url: url,
type: “POST”,
contentType: “application/json;odata=verbose”,
data: JSON.stringify(item),
headers: headers,
success: function(data) {
success(data);
},
error: function(data) {
failure(data);
}
});
},
GetItemTypeForListName: function(name) {
return “SP.Data.” + name.charAt(0).toUpperCase() +
name.split(” “).join(“_x0020_”).slice(1) + “ListItem”;
}
}
}
[/SyntaxHLNumbers]