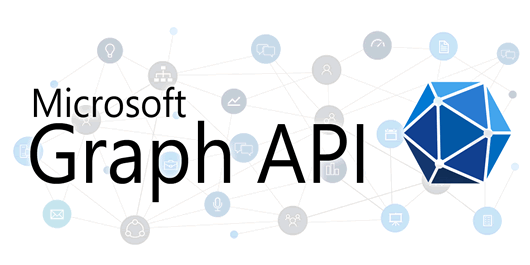
I couldn’t find any examples online on how to get all events in a calendar programmatically using Microsoft Graph and C#. The only example I could find would return 10 items, being the page limit.
In order to save others time, I decided to post the way I managed to do it, using Skip. This code will make a lot of queries, so if you have a better way please email me and I’ll update this post.
No more talk. Here’s the code:
public async Task<List<Event>> GetEvents(string userId, string calendarId)
{
GraphServiceClient graphServiceClient = CreateGraphServiceClient();
var events = new List<Event>();
var isNext = true;
var page = 0;
do
{
var results = (await graphServiceClient.Users[userId].Calendars[calendarId].Events.Request().Skip(page).Top(10).GetAsync());
isNext = results.NextPageRequest != null;
page += 10;
events.AddRange(results.ToList());
} while (isNext);
return events;
}